Ready to zoom in, zoom out and pan around your SVG? An often overlooked technique with SVG animation is animating the viewBox attribute. This can often be a good way to focus on a particular element of your SVG and it’s a little easier than trying to scale and center things.
The SVG layout
For this tutorial, I’m using a simple 800 x 800 SVG with 16 squares.
<!-- Empty SVG --> <svg id="demo" xmlns="http://www.w3.org/2000/svg" width="800" height="800" viewBox="0 0 800 800"></svg>
The 16 squares and numbers are generated with a loop and appended to the SVG. If you’re not too familiar with JavaScript, don’t focus on that part of the demo as it has nothing to do with animating the viewBox. I just didn’t want to manually create a bunch of squares. What we end up with is this:
See the Pen Animate viewBox Basic v1 by Craig Roblewsky (@PointC) on CodePen.
Note: I do have a post about dynamically creating elements if you’d like to know more.
The coordinates
Starting in the upper left corner, you can see the coordinates for this demo. Each square is 200 x 200 units. The initial viewBox is set to “0 0 800 800” and shows us the entire SVG.
The viewBox takes 4 values: “<minX> <minY> <width> <height>”. What does that mean exactly? Think of it like this: You want your viewBox to start at an x/y coordinate (minX, minY) and you want it to extend a certain width/height. Next, we’ll take a look at a real world example.
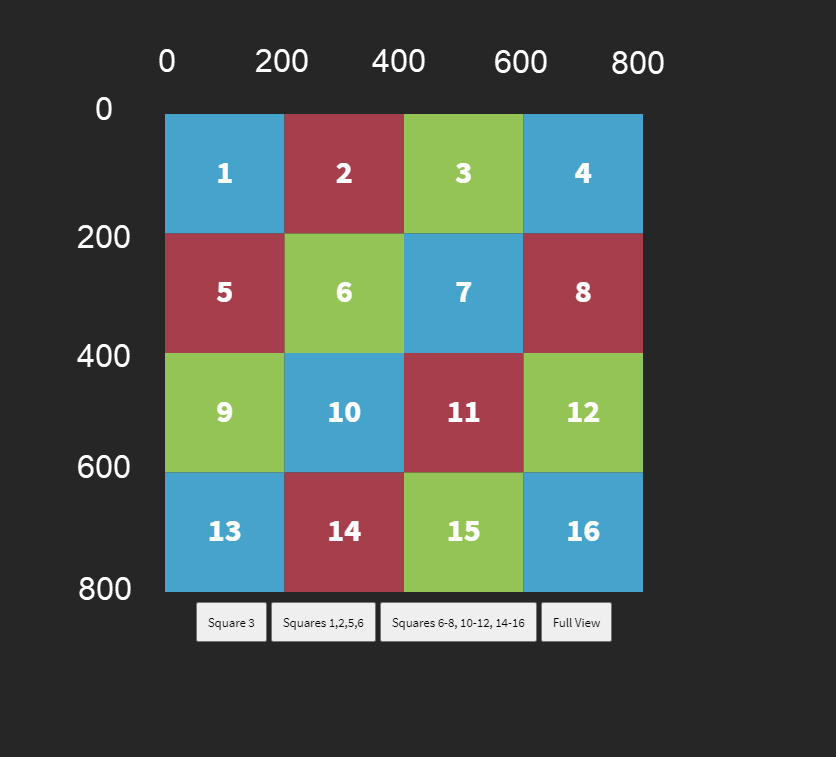
Determine your zoom
Say we want to zoom the SVG viewBox to the ‘3’ square. We can see that the square starts at x:400 and y:0. Your first thought may be to use the coordinates and write a viewBox like this “400 0 600 200”. If so, you’d have the starting coordinates correct, but the width would be 3 times what we need.
Width/height — not ending coordinates
To zoom to the ‘3’ square, you want a viewBox of “400 0 200 200”. The 400,0 starting point is obvious but remember, we only want the width and height. In this case, we know the square is 200 x 200 so those are the values we need for this single square zoom.
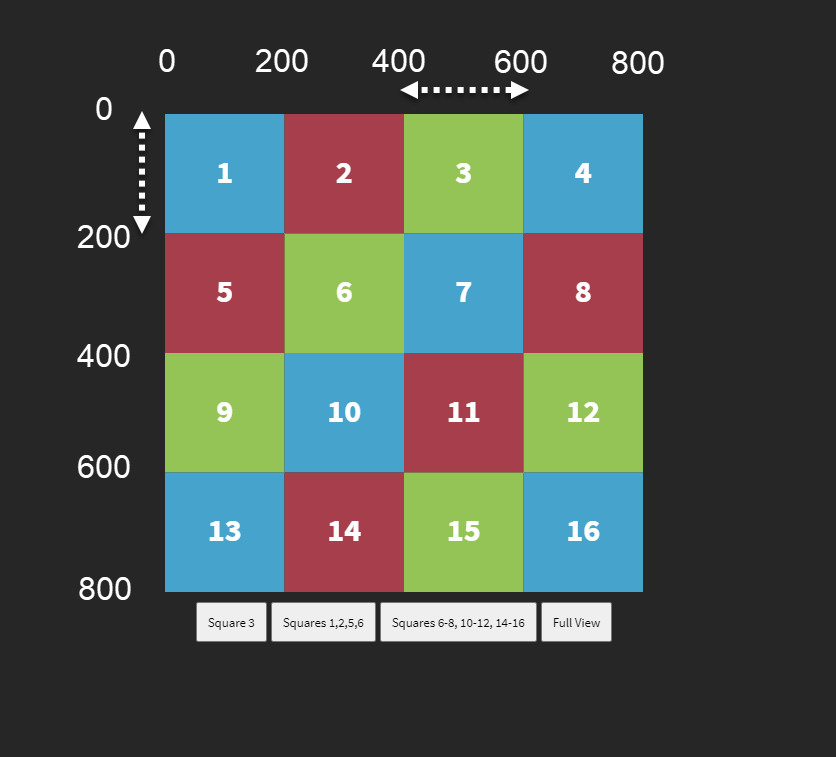
Another example
Let’s say we want to zoom to the 3 x 3 grid of squares in the lower right quadrant of the SVG (6-8, 10-12, 14-16). We can see that group starts at x:200 and y:200. We know each square is 200 x 200 and we have 3 squares by 3 squares. The viewBox is written as “200 200 600 600”.
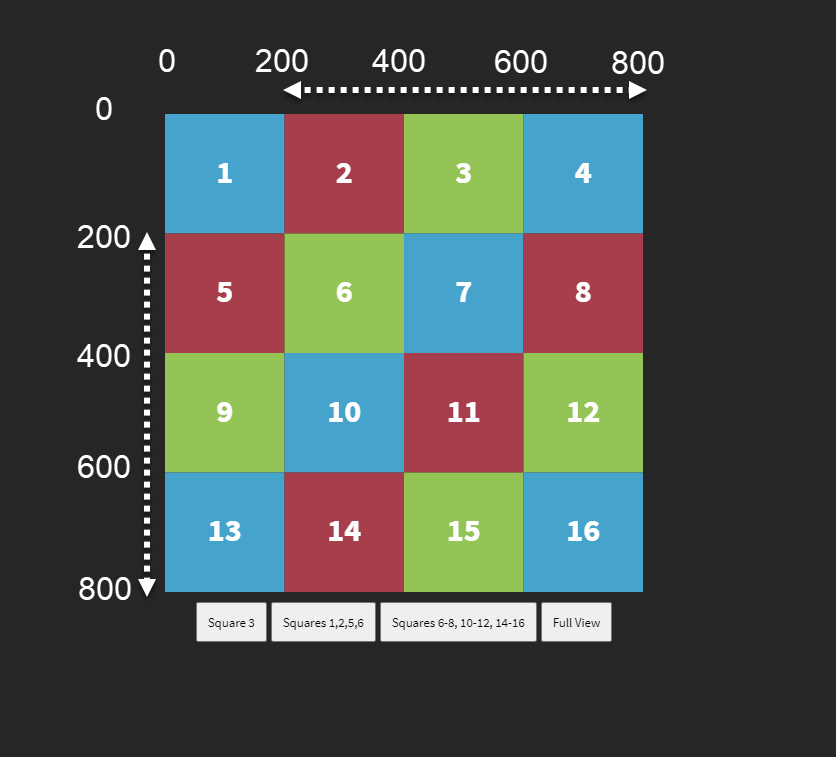
SVG coordinates are not page coordinates
It’s important to understand zooming the viewBox uses the SVG coordinate system. The SVG could be scaled down to 100 x 100 or up to 10,000 x 10,000 on the page. It doesn’t matter. The full viewBox is still 800 x 800 for this demo.
The animation code
Let’s get to the fun part. I’ve added 4 buttons with a data-view attribute matching the viewBox values we discussed above, plus a couple more. Notice the ‘Full-view’ button has the SVG’s original viewBox of “0 0 800 800”.
<div class="buttonWrap"> <button data-view = "400 0 200 200">Square 3</button> <button data-view = "0 0 400 400">Squares 1,2,5,6</button> <button data-view = "200 200 600 600">Squares 6-8, 10-12, 14-16</button> <button data-view = "0 0 800 800">Full View</button> </div>
Each button is given a listener and when clicked, it calls the animateViewBox() function. You then get the attribute and animate the viewBox attribute to the new value.
let targets = gsap.utils.toArray("button"); targets.forEach((obj) => { obj.addEventListener("click", animateViewBox); }); function animateViewBox() { let moveTo = this.getAttribute("data-view"); gsap.to(demo, { duration: 1, attr: { viewBox: moveTo }, ease: "power3.inOut" }); }
The final result
See the Pen Animate viewBox Basic v2 by Craig Roblewsky (@PointC) on CodePen.
Final thoughts
You can see how powerful and fun the viewBox can be for your animations. I’ve hard coded the values in the four buttons, but you can certainly create a more dynamic animation and controls.
You can also trigger other animations when the zoom arrives at certain sections of the SVG. Lots of fun stuff can be achieved. I’ll save those additional features for another tutorial.
Did you pick up a new ‘view’ of SVGs today? See what I did there? I’m hilarious. Well… maybe not, but I do hope you found some of the info helpful. Until next time, keep your pixels movin’.