If you’ve not yet used the MotionPath plugin from GreenSock, here’s a quick tutorial to show you the basics. It has money and a piggy bank. What more could you want?
Vector Files
Jumping into Adobe Illustrator, I set up a background rectangle so I didn’t get any coordinate surprises. Next, I lined up the piggy bank at the bottom and drew three paths with the pencil tool.
You can see the paths (1,2,3 in figure 1). Nothing too fancy or complicated, but a couple of things to note. See how all the paths end at the bottom of the bank? (#4 in figure 1) That allows the money to travel all the way into the bank. If you end the paths right at the top slot, the money wouldn’t travel far enough along the path.
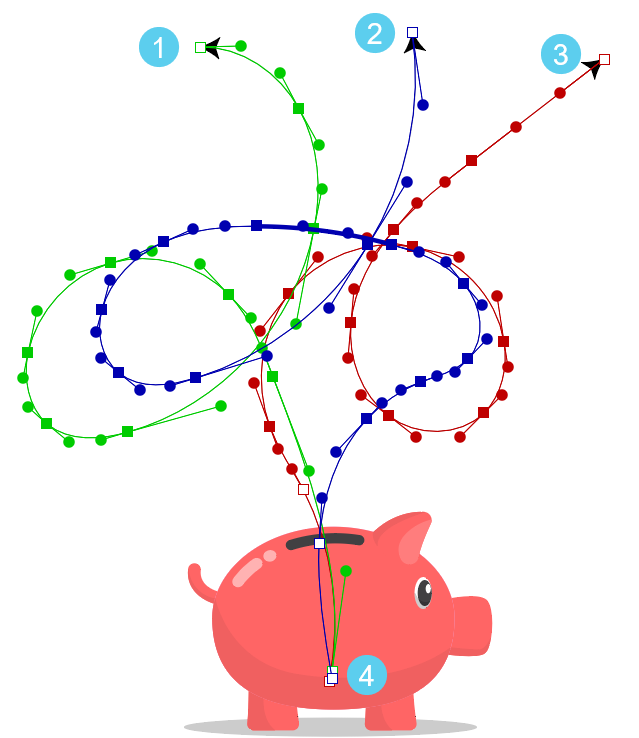
Please note I also added a temporary arrowhead so I could be certain that the start of the paths would be at the top.
The clip-path
The next thing we need is a clip-path. This is just a rectangle I drew out down to the top of the piggy bank slot. Then, I duplicated the slot path and merged it with the rectangle. That merged path will be the clip-path for the flying money. You can see the clip-path in figure 2. I lowered the opacity of the piggy bank to more easily see the extra slot path merged into the rectangle.
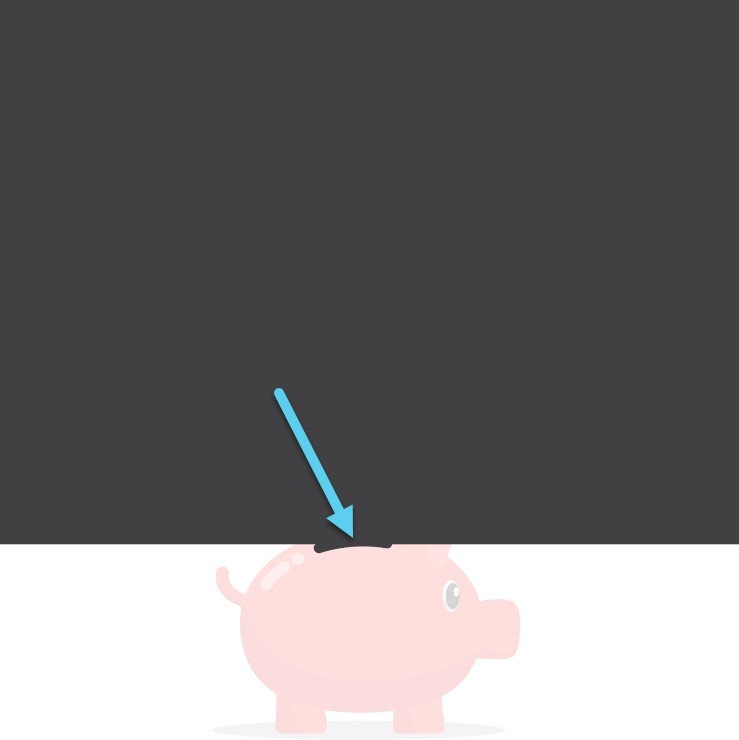
The flying money
The money group is just a few paths and I duplicated it twice. It makes no difference where they are positioned as the MotionPath will align them for us. Those three groups are in a parent group, which is clipped by the clipPath.
<clipPath id="theClipPath"> <path d="M800,0H0V586H341.16a5.15,5.15,0,0,0-1.91,5.92l0,.1a5.24,5.24,0,0,0,6.5,3.24,154.4,154.4,0,0,1,70.59-5,5.25,5.25,0,0,0,6-4.13l0-.1v0H800Z" fill="#414042" /> </clipPath> <g id="money" clip-path="url(#theClipPath)"> <!-- groups of paths --> </g>
The animation code
If you only have one target and one motion path, the animation won’t involve a loop for the setup. In this case, I have three motion paths and three money groups so I used a forEach loop to make the animations.
First, we need to register the plugin and declare a variable for the motionPaths and targets. I’m using a handy utility method from GreenSock called utils.toArray() for this.
gsap.registerPlugin(MotionPathPlugin); let motionPaths = gsap.utils.toArray("#motionPaths path"); let targets = gsap.utils.toArray("#money g");
Start the loop
The first part of the loop creates a unique duration and delay for each target. We also create a timeline for each target and path. The duration and delay come from another handy utility method from GreenSock called simply gsap.utils.random(). Using a different duration and delay for each money group prevents each one from playing and looping at the exact same time.
targets.forEach((obj, i) => { let dur = gsap.utils.random(6, 8); let delay = gsap.utils.random(0, 1); let tl = gsap.timeline({ defaults: { ease: "sine.in" }, repeat: -1, delay: delay });
Second part of the loop
Now comes the fun part. The first tween in each timeline scales the money group up from 0. The duration for this is only half of the total duration variable because we want it to reach full size around the halfway point of the motion path.
The second tween aligns each money group to each motion path. Remember, the money and the motion paths are in arrays so they are easily accessed via the index in the forEach loop.
The autoRotate can be set to true (or not used at all) but in this case, the money needed to be rotated 90 degrees otherwise it would animate along the path sideways. Feel free to adjust that in the CodePen demo and see the difference. Just change autoRotate to true. The second tween has a position parameter set to 0 so it starts at the same time as the scale tween.
tl.from(obj, { duration: dur / 2, scale: 0}); tl.to( obj, { duration: dur, motionPath: { path: motionPaths[i], align: motionPaths[i], alignOrigin: [0.5, 0.5], autoRotate: 90 } }, 0 ); });
The result
Once we have all that in place, we get a neat little piggy bank with flying money. Please feel free to fork the demo and play with it a bit.
See the Pen Piggy Bank With Motion Path by Craig Roblewsky (@PointC) on CodePen.
Final thoughts
That’s just the very beginning of what GreenSock’s MotionPath plugin can do. In the future, I’ll have new tutorials that will take a deeper dive into additional features.
I hope you enjoyed this little primer and picked up an idea or two. Until next time, keep your pixels movin’.